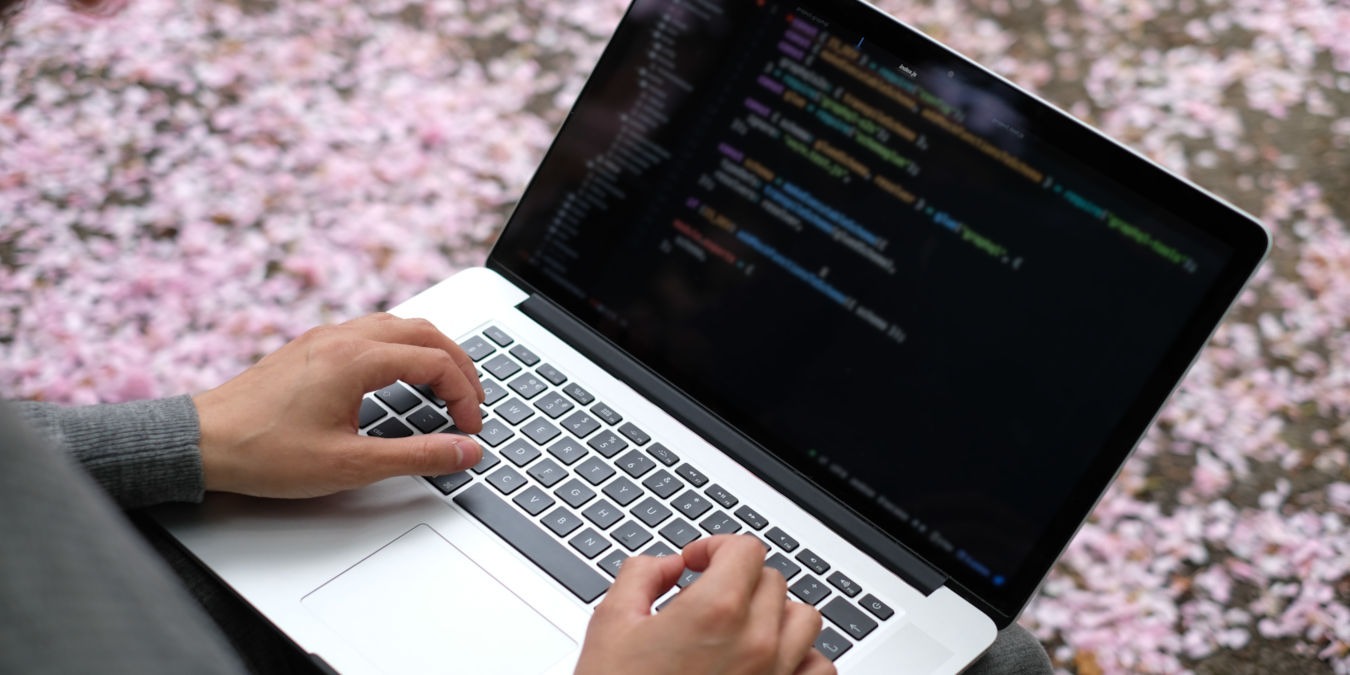
Bash allows you to map whole strings of text to single variables, simplifying its use and script writing. How do they work, and how can you use them? Let’s find out.
What’s a Bash Variable?
Variables are easy-to-remember names that can contain different alphanumeric values. They’re useful, as they allow the same function to be applied on different values, without having to rewrite a script/piece of code. They also make writing the script/piece of code easy, since instead of dealing with individual values, you can use the same name for all of them.
Real-Time Variables
Bash allows the use of variables. You can create variables on the fly and reuse them during your current Bash session. They can assist your use of Bash in many different ways and will be gone after the current session ends.
For example, let’s say you’re visiting a bunch of sites. You could be doing research or scraping data and create the following variable:
sitea=https://www.maketecheasier.com
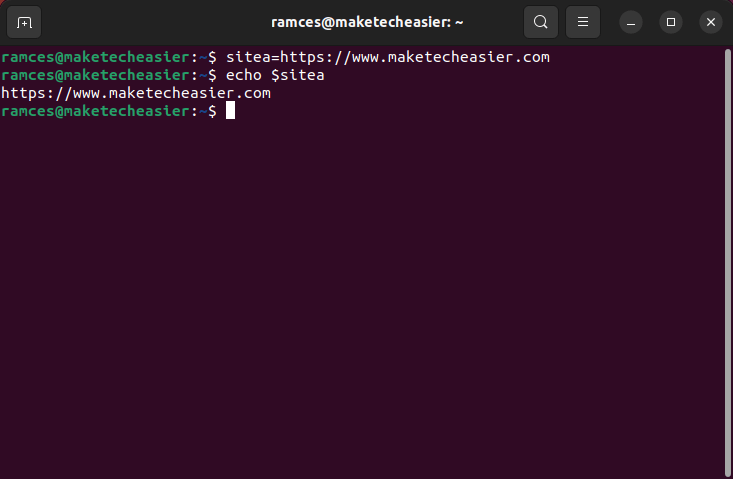
After that, if you wanted to visit our site with Firefox, you could just type:
firefox $sitea
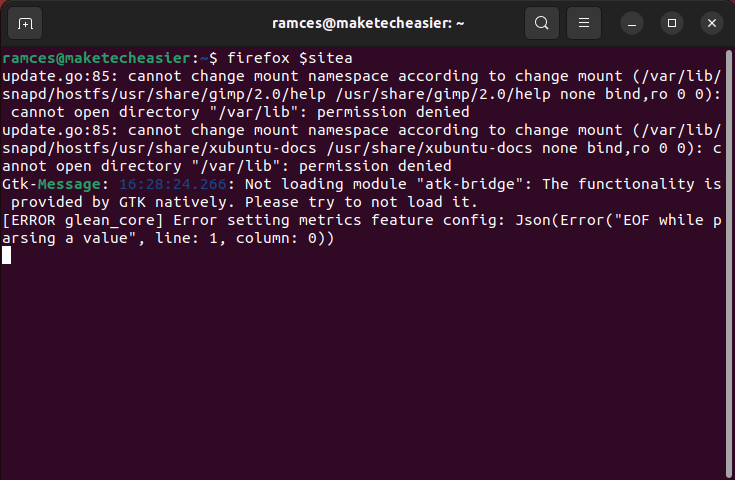
Much easier – and more readable. The $sitea
variable would remain mapped to the site until you either changed its contents manually or the Bash session ended. And, of course, you can create more variables, such as siteb
, sitec
, and sited
.
When setting up new variables, you can use any names you wish and store any alphanumeric strings inside them. Do keep in mind, though, that they’re case-sensitive by default. Thus, $sitea
would not be the same as $SiteA
. Also, note that you should use quotation marks when storing strings with special characters inside them (including spaces).
Tip: for those who are new to Bash, get started with these basic Bash commands.
Variables in Scripts
Variables in Bash are more useful when writing scripts, as they allow you to write a single script, which can then iterate through different strings or act on customized pieces of data. Let’s say that you’re writing a script that everyone could use on their computer, but each time would display a personalized greeting. Without variables, you’d have to write a different version of the script for each user. With variables, you keep the script the same and only change the user’s name.
Such a script would look something like the following:
#!/bin/bash username=Ramces echo $username
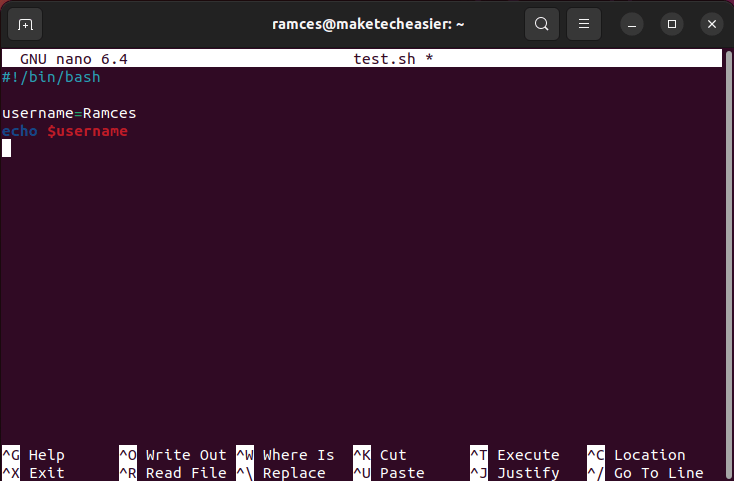
The above example may seem redundant; however, as the complexity of the code increases, variables become indispensable. A script could be hundreds or thousands of lines long and contain the user’s name in different spots. To better understand it, consider the following somewhat different script:
#!/bin/bash username=Linus echo Hello $username. This is a simple script I wrote that will repeat your name - that is, $username - often as an actual example of how to use Bash variables. I hope it will make it easier to understand how to use variables to improve your Bash workflow, $username. In this case, the variable username is mapped to your name. Whenever Bash runs into it, it replaces it with $username.
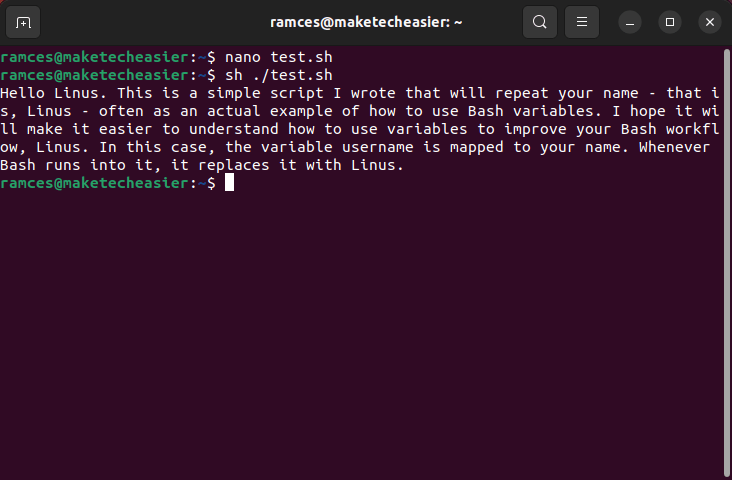
The above script will use the name defined as the username
variable to complete the text. If using the actual user’s name, you’d have to type it four times, then do the same for the next user, and another four times for the next. Again and again. By assigning it to a variable, you only have to change it once for each user, and every mention of the user’s name in the text will be updated.
Good to know: other than variables, you can also use the for loop in Bash.
Permanent Bash Variables and Aliases
We saw how you can temporarily set variables and how, for something more permanent, you can include them in your own scripts. You can also permanently set variables in Bash by editing the “~/.bashrc” file.
- Open the file “~/.bashrc” in your favorite text editor.
nano ~/.bashrc
- We suggest you begin with a test run, only adding a single variable, so that you’ll know where to look if the process doesn’t work out. Move to the end of the file and, in a new line, add your variable.
myname="Ramces Red"
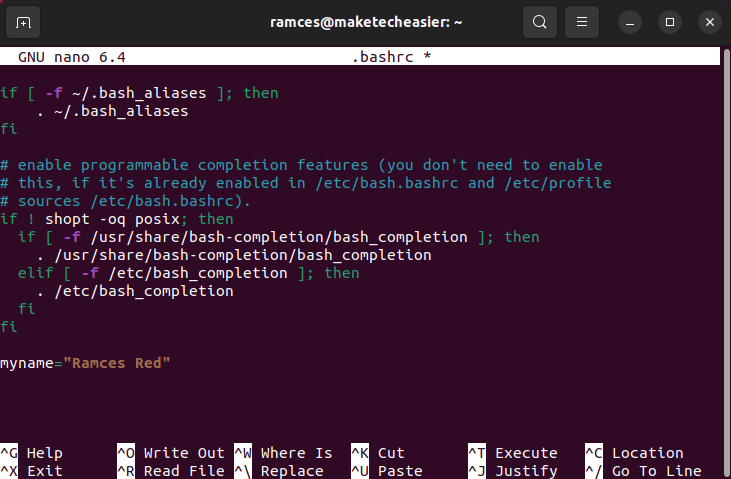
- Save your file and exit the editor. The tweaks won’t be applied immediately. Enter the following in your terminal for it to take effect:
source ~/.bashrc
- Make use of the newly set variable in your Bash session:
echo $myname
You can set up as many variables as you would like and vastly simplify your daily adventures in Bash.
For an extra boost in productivity, it’s also worth setting up a different type of variable: aliases. Unlike typical variables, which are mapped to data you can use in commands, aliases are used instead of actual commands.
Just as you can use a simple-to-remember variable to hold long strings of text, you can use aliases as easy alternatives to complex commands. You can find out more about them here, where we turn a whole 7zip compression command into a two-character alias.
Lastly, even if you’ve permanently set a variable in .bashrc, you can reassign a different value to it temporarily, as we saw before. The variable will present the new content until the current Bash session ends (after logging out or restarting) or you re-source the .bashrc file.
Creating Arrays
While most Bash users think of variables as a simple link between a piece of data and a label, it is also possible to use them as a marker for arrays. These are a type of data structure that can store multiple values in a numbered index format. To create an array, follow the steps below:
- Create an empty shell script:
nano array.sh
- Use the
declare
subcommand to initialize the Bash array in the current shell process.
declare -a myarray
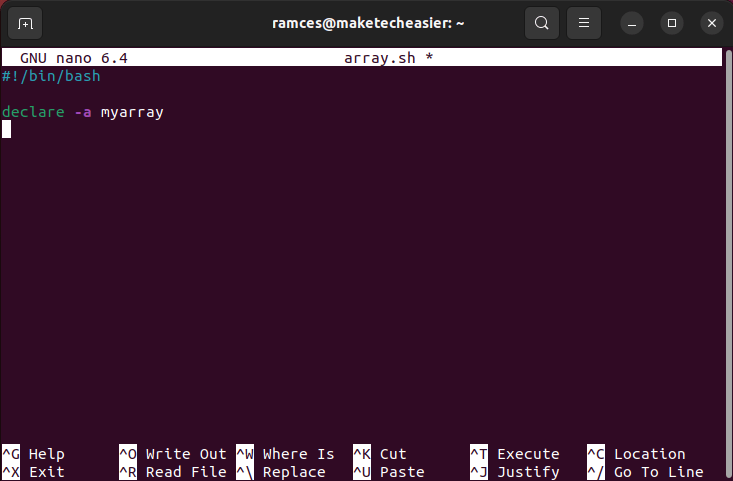
- Populate your new array by using the built-in Bash assignment operator. For example, the following line of code creates an array with five elements inside it:
myarray=([0]=hello [1]=maketecheasier [2]=its [3]=an [4]=array)
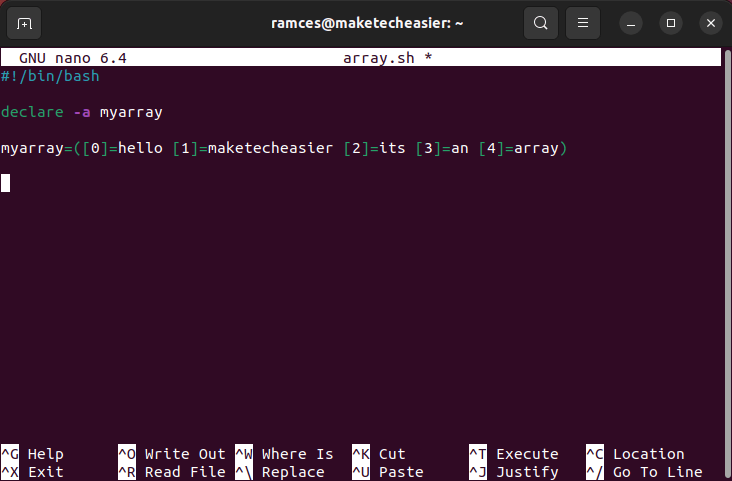
- Test whether your new array works by using the
echo
command to pull data from it:
echo ${myarray[1]}
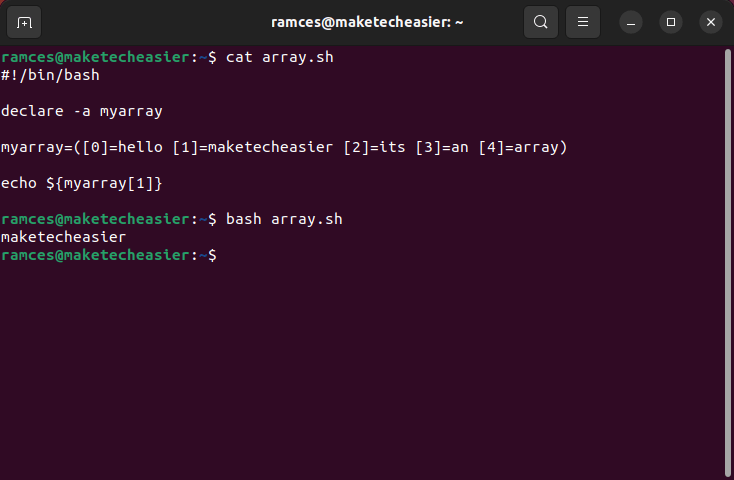
You can also stream the contents of an array by using the “*” operator:
echo ${myarray[*]}
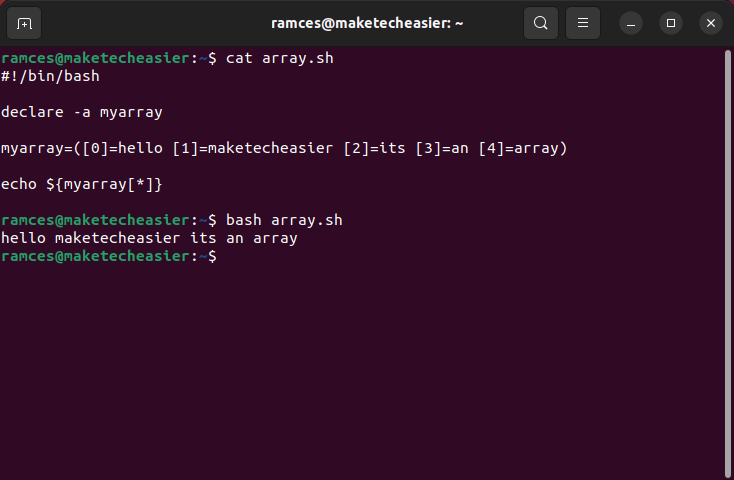
Executing Subshell Variables
One of the biggest features of Bash is its ability to create subshells, where programs can run as if they are basic functions. For example, writing ls -a
in a shell script and running it will still print the contents of the current working directory.
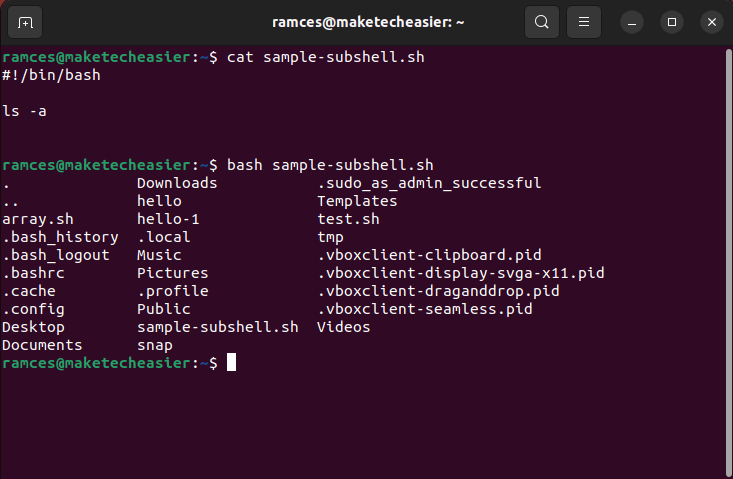
While this may look limiting, Bash extends this by allowing you to store the output of these programs inside variables. This allows you to use them as the input for other programs inside your script.
- To use subshell variables, first create an empty shell script:
nano subshell.sh
- Write the following boilerplate code inside your new shell script:
#!/bin/bash mysubshell=$(x) echo "The output of the variable is $mysubshell"
- Replace the “x” with the command that you want to run and store the output. For example, the following will get the contents of the world.txt file and replace the word “world” with “maketecheasier.”
mysubshell=$(sed s/world/maketecheasier/g world.txt)
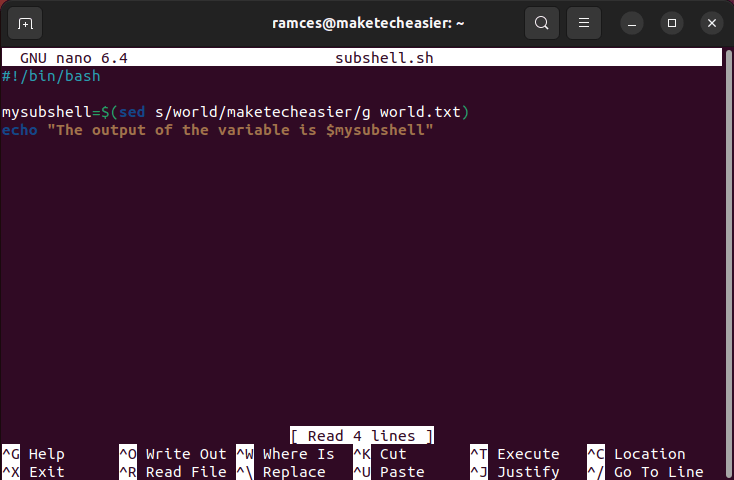
- Test your new subshell variable by running your new script:
chmod u+x ./subshell.sh bash ./subshell.sh
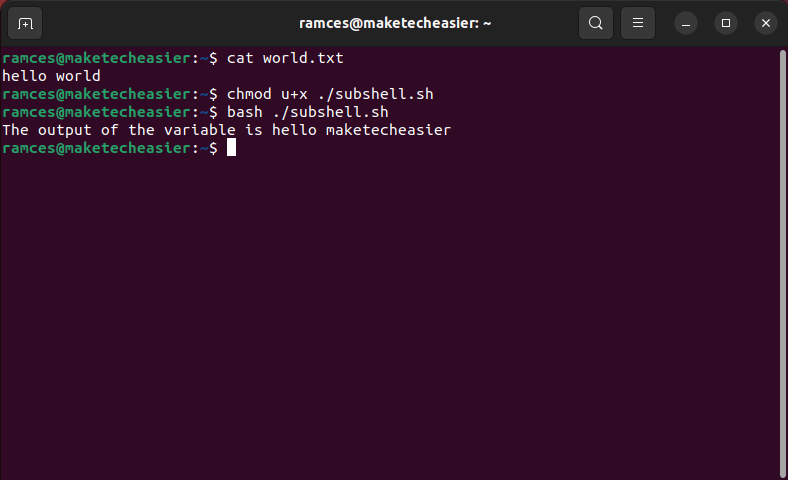
FYI: in addition to variables, these are the special characters you should know in Bash.
Substring Manipulation
Aside from storing and retrieving data, you can also modify the contents of variables. Bash has a set of operators that you can attach at the end of every variable, which will allow you to fine tune your scripts without calling any additional programs.
- Create an empty shell script:
nano substring.sh
- Write the following boilerplate code inside your empty script:
#!/bin/bash mysubstring=hello.txt.old echo "My filename is: ${mysubstring}"
- Basic substring manipulation comes in three forms. First, the “#” character accepts a regex pattern that the shell will remove at the beginning of the variable:
#!/bin/bash mysubstring=hello.txt.old echo "My filename is: ${mysubstring#*.}"
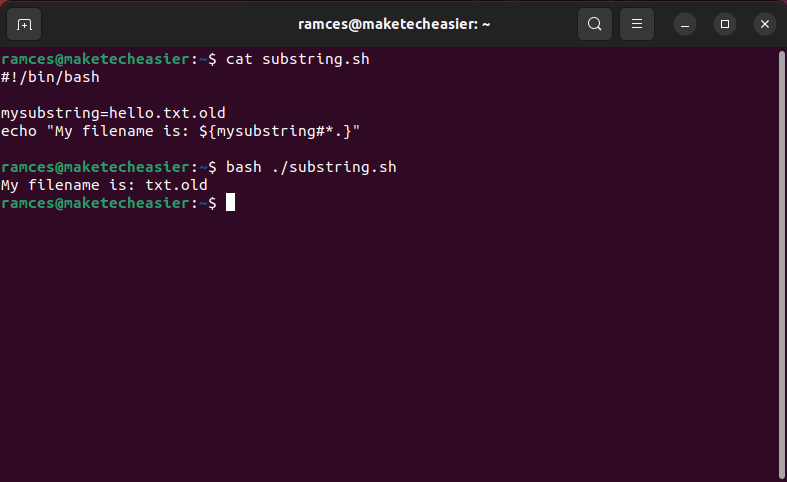
- Second, the “%” character also accepts a regex pattern that the shell will remove at the end of the variable:
#!/bin/bash mysubstring=hello.txt.old echo "My filename is: ${mysubstring%.*}"
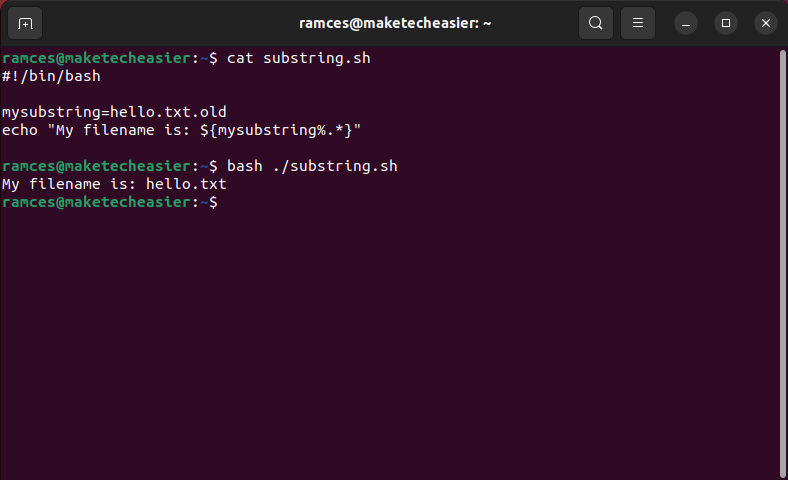
- Bash also provides a way to selectively modify the contents of a variable. Similar to the
sed
program, you can use the “/” character to find and replace any string inside a variable:
#!/bin/bash mysubstring=hello.txt echo "My filename is: ${mysubstring/hello/maketecheasier}"
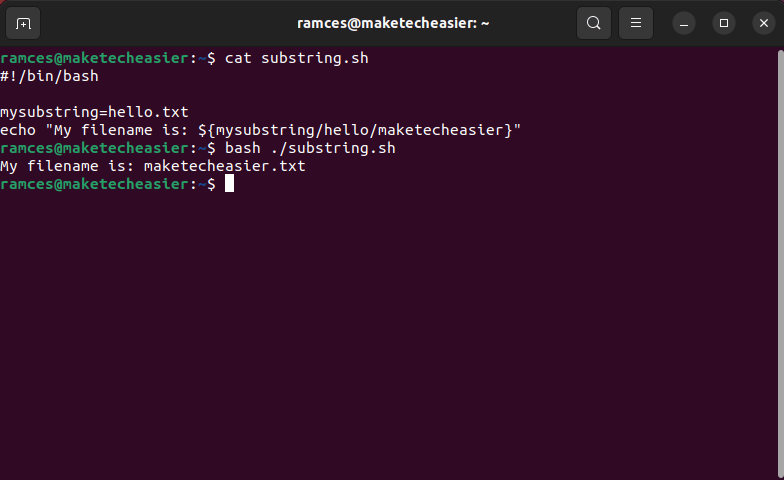
Good to know: regular expressions are an important tool in every Linux user’s toolkit. Learn how to use it effectively through our easy to learn cheatsheet.
Frequently Asked Questions
Is it possible to run a shell script inside a variable?
Yes, by writing the full path of the script that you want to run. For example, running $(/home/ramces/.scripts/sample.sh)
will create a Bash subshell and run the “sample.sh” script from my home directory.
Can you remove a variable while a script is running?
Yes. You can remove a variable from a running shell process by inserting the unset
subcommand followed by the name of the variable in your script. It is important to note that running this command will not remove any variables that run outside the current shell process.
Can you use a permanent variable inside an external shell script?
Yes. It is possible to refer to the value of a variable that was declared outside of the current shell process. However, you also need to be wary when using external variables, as it is easy to temporarily overwrite them.
Image credit: Unsplash. All alterations and screenshots by Ramces Red.
Our latest tutorials delivered straight to your inbox